Python to C#: The Path to Becoming a Software Developer
2024-12-12 Back to posts
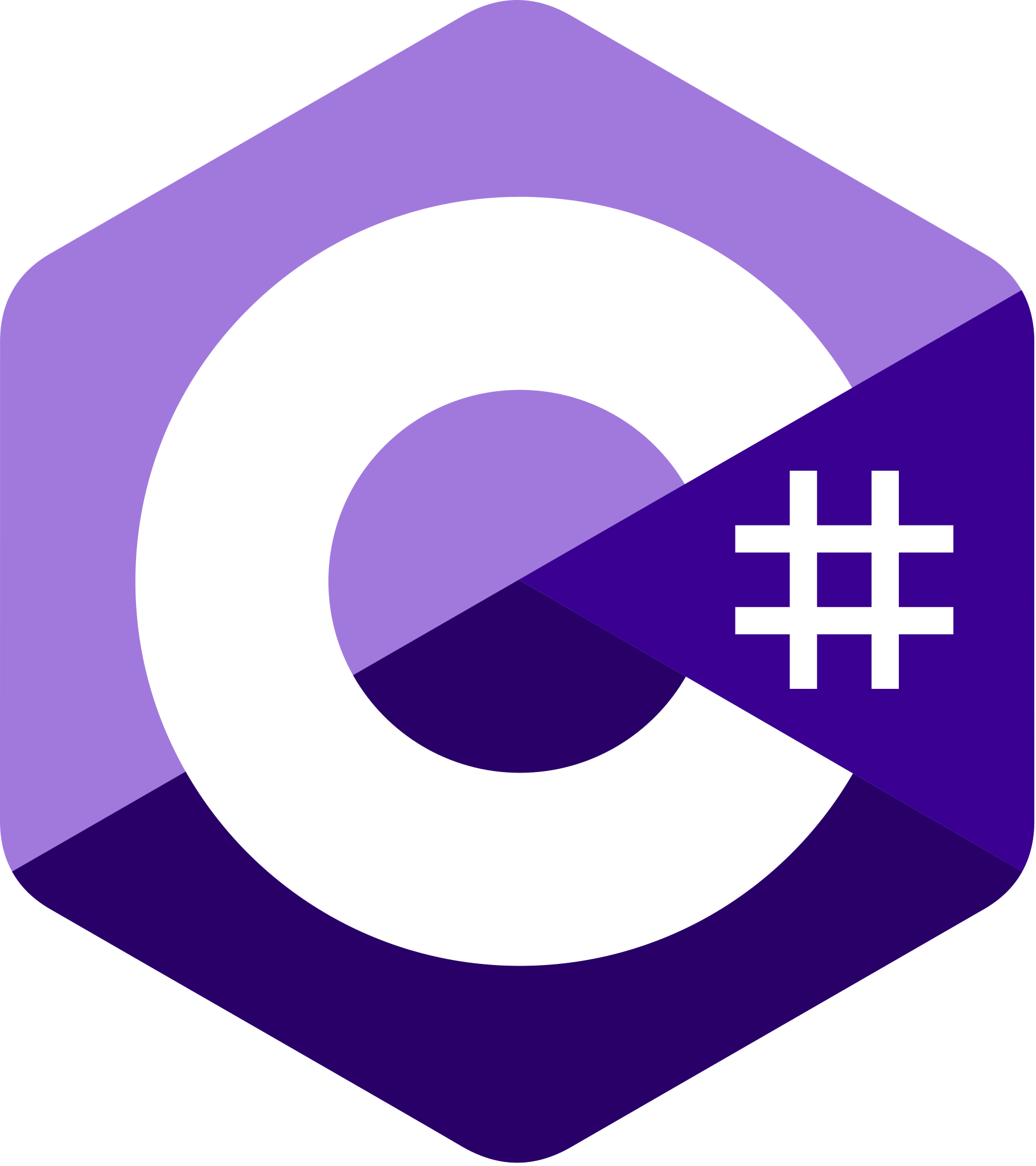
Python to C#: My Path to Becoming a Junior Developer
Starting a new role as a Junior Application Developer is exciting.
When I accepted the position, I knew I had to level up my skills in C#.
My experience with Python gave me a solid foundation, but diving into a new language on a tight timeline is its own kind of challenge.
Here’s how I’m tackling it.
Building Familiarity with C# Basics
Switching from Python to C# initially felt overwhelming.
Python’s dynamic and loosely typed nature is forgiving, while C# demands strict attention to types and structure.
But this structure is exactly what makes C# so powerful for building enterprise-level applications.
To get started, I focused on understanding the syntax and core concepts:
- Variables and Data Types: Unlike Python, C# requires explicit declarations, so I spent time practicing with
int
,string
, andbool
to get comfortable.
Here’s an example:
// Declaring variables in C#
int age = 25;
string name = "Nick";
bool isJuniorDeveloper = true;
Console.WriteLine($"Name: {name}, Age: {age}, Junior Developer: {isJuniorDeveloper}");
- Object-Oriented Programming (OOP): While Python supports OOP, C# leans heavily into it. Concepts like classes, inheritance, and interfaces are central to writing clean, modular code.
For example:
// Simple C# class and object creation
class Developer {
public string Name { get; set; }
public string Role { get; set; }
public void Introduce() {
Console.WriteLine($"Hi, I am {Name} and I work as a {Role}.");
}
}
Developer dev = new Developer { Name = "Nick", Role = "Junior Developer" };
dev.Introduce();
One thing I learned quickly: C#‘s type safety saves headaches later by catching errors at compile time instead of runtime (looking at you, JavaScript).
Leveraging Resources for Learning
I needed to make my learning efficient, so I turned to resources designed for hands-on practice.
Some of the tools I’ve found invaluable include:
-
Microsoft Documentation: C#‘s official docs are thorough and up-to-date. I’ve used them to dive deep into topics like LINQ and async programming.
-
Pluralsight and YouTube Tutorials: Video tutorials help break down complex topics into digestible pieces.
-
Mini Projects: The best way to learn is by doing. I’ve started building small apps, like a console-based to-do list, to put concepts into practice.
For example:
// Simple to-do list program
List<string> tasks = new List<string>();
while (true) {
Console.WriteLine("Enter a task (or 'exit' to quit):");
string input = Console.ReadLine();
if (input.ToLower() == "exit") break;
tasks.Add(input);
Console.WriteLine("Current Tasks:");
tasks.ForEach(task => Console.WriteLine($"- {task}"));
}
Adopting New Patterns
Coming from Python, I was used to quick prototyping and flexibility.
C# introduced me to new patterns:
-
Dependency Injection: Learning to decouple code components.
-
Asynchronous Programming: C# makes async development a first-class citizen, and grasping
async/await
has helped me write more efficient, non-blocking code. Here’s a small example:
// Example of async programming in C#
using System.Net.Http;
async Task FetchDataAsync() {
HttpClient client = new HttpClient();
string data = await client.GetStringAsync("https://api.example.com/data");
Console.WriteLine(data);
}
await FetchDataAsync();
These patterns may feel rigid at first, but they promote maintainability---a key trait in development.
Setting Milestones
With limited time, I’ve broken my preparation into milestones:
-
Master Core Syntax: This included
types
,loops
, andconditionals
. -
Understand OOP: I’ve been practicing
encapsulation
andinheritance
by building small projects. -
Explore Frameworks: C# is deeply tied to .NET, so I’ve started exploring
ASP.NET Core
for web development andWPF
for desktop apps. -
Build a Capstone Project: To tie everything together, I’m working on a
CRUD
application that leverages aSQL database and ASP.NET Core
.
Reflections on the Transition
Transitioning from Python to C# has been rewarding.
While Python prioritizes simplicity, C# shines in structured, large-scale applications.
Each language has its strengths, and I’ve come to appreciate how learning both expands my toolkit.
Conclusion
This journey isn’t just about learning a new language; it’s about preparing to contribute meaningfully as a Developer.
If you’re also navigating a new tech stack, take it one step at a time and just breathe.
Written By Nick Stambaugh